Introduction
In the dynamic world of web development, React stands out as a powerful JavaScript library that enables developers to build high-performing, interactive user interfaces. Whether you’re a seasoned developer or a beginner eager to dive into React development, running a React app locally is a fundamental skill you’ll need to master. This blog post provides a comprehensive, step-by-step guide on how to run a React app locally, ensuring you have all the necessary tools and knowledge to get your application up and running smoothly on your own machine.
Understanding how to set up and run a React app locally is crucial for development and testing, allowing you to see and interact with your changes in real-time. By the end of this guide, you’ll not only be equipped to launch a basic React app but also understand the workings of the React development server and how to troubleshoot common issues that may arise during the process.
Table of Contents
Prerequisites for Running a React App Locally
Before you can dive into the world of React and start running applications on your local machine, there are several prerequisites you need to meet. These requirements are essential as they form the backbone of your development environment, ensuring that your React app can be installed, executed, and managed effectively. Here’s what you need to prepare:
- Basic Knowledge of JavaScript and Web Technologies: Familiarity with JavaScript, HTML, and CSS is crucial since React is a JavaScript library. Understanding these technologies will help you troubleshoot and better understand how React works within web pages.
- Node.js and npm (Node Package Manager): React applications rely on Node.js to run JavaScript code outside the browser. npm is used to manage the libraries and dependencies that your React app will need. Ensuring you have the latest stable version of Node.js and npm installed on your computer is vital for a smooth setup and execution process.
- A Suitable Code Editor: While you can write code in any text editor, using a code editor like Visual Studio Code, Sublime Text, or Atom can enhance your coding experience with features like syntax highlighting, auto-completion, and direct integration with Git.
- A Command Line Interface (CLI): Comfort with using the command line is beneficial for running npm commands, starting the development server, and interacting with your React app’s environment.
- Internet Connection: An active internet connection is necessary to download React and its dependencies.
Once these prerequisites are in place, you’re ready to move on to installing the core technologies that will power your React app. This setup is the first technical step in your journey to running a React app locally.
Installing Node.js and npm
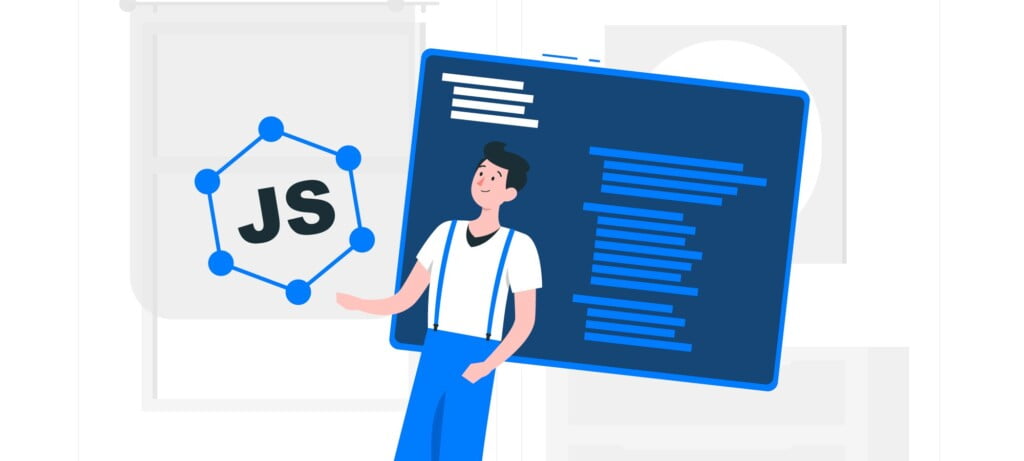
To start building and running your React applications, you first need to install Node.js and npm (Node Package Manager). Node.js is the runtime environment required to run JavaScript code outside the web browser, and npm is the package manager that facilitates the installation and management of JavaScript libraries and dependencies. Here’s how to install both on your system:
- Download Node.js:
- Visit the official Node.js website (nodejs.org).
- Select the version recommended for most users (this ensures stability and compatibility).
- Download the installer for your operating system (Windows, MacOS, or Linux).
- Install Node.js:
- Run the downloaded installer.
- Follow the installation prompts, ensuring that npm is selected for installation along with Node.js.
- Complete the setup by following the on-screen instructions, which typically involve clicking “Next” until the installation begins and finishes.
- Verify Installation:
- Open your command line interface (CLI).
- Type
node -v
and press enter to check the Node.js version, which confirms its installation. - Type
npm -v
to check the npm version, verifying that npm is also installed correctly.
With Node.js and npm installed, you have the necessary tools to create a React application and manage its dependencies.
Setting Up a React Project
Once Node.js and npm are installed, the next step is setting up a new React project. This can be done easily using the Create React App command-line utility, which sets up everything you need for a React application. Here’s how to do it:
- Open your CLI and navigate to the directory where you want your project:
- Use
cd
command to change directories in your CLI.
- Use
- Create a New React Application:
- Run the command
npx create-react-app my-app
, replacingmy-app
with your desired project name. This command pulls the Create React App builder from npm and sets up a new React project with all the default configuration and dependencies. - Wait for the process to complete—it installs several dependencies and might take a few minutes depending on your internet speed.
- Run the command
- Navigate into your project directory:
- Use
cd my-app
to move into your project folder.
- Use
- Explore Your React Project:
- Once inside your project directory, you can open the entire folder in your code editor to see the structure of a basic React application, including the
src
folder where you’ll primarily work, and thepublic
folder which contains static assets.
- Once inside your project directory, you can open the entire folder in your code editor to see the structure of a basic React application, including the
By completing these steps, your basic React project setup is ready. You now have a local development environment where you can start building your React application.
Running Your React App Using npm
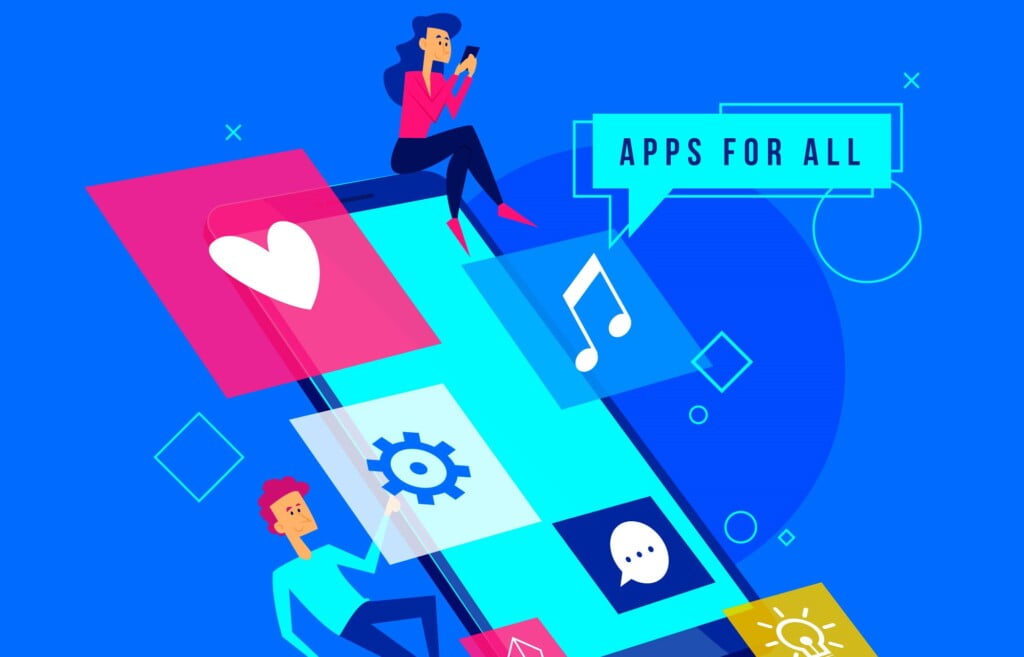
To see your React application in action, you need to run it using npm, which will start a development server on your local machine. Here’s how to run your app:
- Start the Development Server:
- In your CLI, ensure you are in your project directory.
- Run the command
npm start
. - This command compiles your React code and opens your application in a web browser automatically.
- Access Your App:
- By default, Create React App will run your application on
http://localhost:3000
. You can open a web browser and navigate to this address if it doesn’t open automatically. - You will see your React app’s default welcome screen, indicating that everything is running correctly.
- By default, Create React App will run your application on
- Make Changes and See Them in Real-Time:
- Any changes you make to your React code in the
src
folder will automatically refresh the page on the browser, allowing you to see updates in real-time.
- Any changes you make to your React code in the
Using npm to run your React app locally is a straightforward process that leverages the power of the React development server, which we will discuss next.
Understanding the React Development Server
The React Development Server is a built-in local server provided by the Create React App environment. It serves your React application to the browser during development, allowing for a seamless workflow with instant feedback. Here’s what you need to know about how it operates and enhances your development process:
- Hot Module Replacement (HMR): The development server comes equipped with Hot Module Replacement technology. HMR updates the application in the browser as you edit and save files, without needing a full page reload. This means you can maintain application state between changes, which is especially useful when tweaking the UI or testing interactive features.
- Bypassing Configuration Complexity: With the React Development Server, there’s no need for manual configuration of webpack or other bundlers. The server handles all the complex configuration behind the scenes, so you can focus on writing your React components and logic without worrying about the underlying tools.
- Built-In Optimization: The server optimizes your development experience by providing features like linting and fast refresh. Errors and warnings are displayed directly in the browser, making it easier to spot and fix issues immediately.
- Proxy Support: For development purposes, you can configure the server to proxy API requests to your backend server. This is particularly useful in a full-stack development environment where your React app needs to interact with external APIs that are also under development.
Customizing Your Local Environment
Customizing your local React development environment can further enhance productivity and adapt the setup to your preferences and project needs. Here are some ways to tailor your environment:
- Environment Variables: You can manage different environments (development, production, testing) by setting up environment variables. Create React App supports
.env
files where you can define variables for each environment, such as API endpoints, configuration settings, and more. - Customizing Webpack and Babel: While Create React App comes with a pre-configured setup, you might want to tweak webpack or Babel settings for advanced scenarios. You can ‘eject’ from the default setup by running
npm run eject
. This exposes all configuration files, allowing you to customize as needed. Note that ejecting is a one-way operation—once you do it, you can’t go back. - Adding Styling and Assets: Incorporate CSS frameworks like Bootstrap or Material-UI, or use CSS-in-JS libraries like styled-components for more dynamic styling. Managing assets like images, fonts, and icons efficiently can also be configured to optimize performance and organization.
Troubleshooting Common Issues
Running a React app locally can sometimes lead to common issues. Here are some typical problems and how to resolve them:
- Module Not Found Errors: These errors usually occur when dependencies are not installed correctly. Ensure all your packages are installed by running
npm install
in your project directory. - Port Already in Use: If you get an error stating that the port (default 3000) is already in use, you can change the port by setting the
PORT
environment variable, like so:PORT=3001 npm start
. - Browser Not Refreshing: If changes are not reflecting in the browser, make sure that your browser’s cache is not interfering. You can often resolve this by clearing the browser cache or enabling “Disable cache” in the browser’s developer tools settings when the dev tools are open.
- Version Incompatibilities: Sometimes, new versions of Node.js or npm might not be compatible with certain packages. Check the package documentation for version requirements and consider using a node version manager like
nvm
to switch between different Node.js versions.
Conclusion
Setting up and running a React app locally is a crucial skill for any developer working with this library. By following these detailed steps—from installation to troubleshooting—you can ensure a robust development environment that lets you focus on creating impressive web applications. Whether you are building small personal projects or large-scale enterprise applications, understanding these fundamentals will greatly assist in your React development journey.
Read Our Other Article here…